Introduction
Git is the most commonly used version control tool for coordinating CI/CD work among programmers. Popular code repositories include GitLab, Bitbucket, and others. We have to checkout, add, commit, and push every time we want to update the repo. It is straightforward if the developer tests locally and pushes codes once via Git Bash command line or VS Code plugin. Nonetheless, in my ‘Ansible Tower’ project, I am generating the playbook from a Python web form, which I then have to manually commit to the remote repository. I thought why not integrate the commit and push after the playbook is made automatically.
Step 1 — Install GitPython Library to localhost
GitPython library is needed to interface with Git repositories. This python library provides object model read and write access to the git repository. The requirement is Python >= 3.7
- Windows:
C:\>python3 -m pip install gitpython
orC:\>python -m pip install gitpython
- Linux:
$ pip install GitPython
- macOS:
~ % pip install gitpython
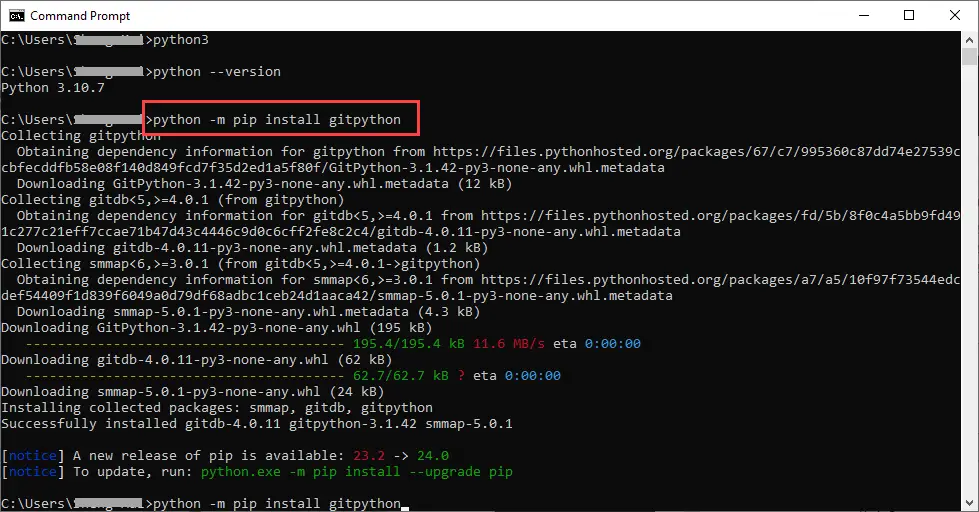
Step 2 — main.py
The main.py script will search for the git root directory in the project folder. The directory path will be returned to repo = git.Repo(git_root)
The block steps in the Git process are Checkout → Add → Commit → Push
# main.py
import git
## A. Find git 'root source' DIR by searching Drive:
git_repo = git.Repo("X:", search_parent_directories=True)
git_root = git_repo.git.rev_parse("--show-toplevel")
print("------------------------")
if __name__ == "__main__":
print(git_root)
print("------------------------")
## B. START: Git process
# Auto from search (A) above
repo = git.Repo(git_root)
# Or manual enter repo path, note in this case, Windows is forward slash (X:/)
#repo = git.Repo('X:/devops-roadmap-master')
## B1. Checkout
origin = repo.remote(name='origin')
# Note: Branch is usually 'main' but can be 'master'
existing_branch = repo.heads['master']
existing_branch.checkout()
## B2. Do some changes and Add -> Commit
repo.git.add(all=True)
print('Files Added Successfully')
repo.index.commit('Initial commit on new branch')
print('Committed successfully')
## B3. Push to the repo
origin.push()
print('Pushed changes to origin')
Step 3 — Test with input.txt and test-sample.jpg
Let us create two dummy files (input.txt and test-sample.jpg) within the same directory. To open the PowerShell window, open Windows Explorer and (left-shift + right-click)
X:\devops-roadmap-master\python-git (X: is my designated USB drive) |-- main.py |-- input.txt |-- test-sample.jpg
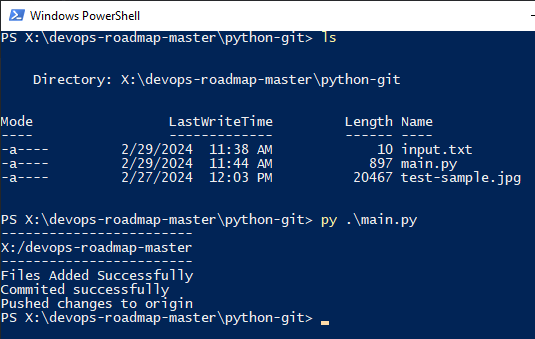
In my case, we will log into the Source Code Repository, which is GitLab. The python-git folder has been added, and any supplementary modifications to its contents will be updated upon execution of main.py.
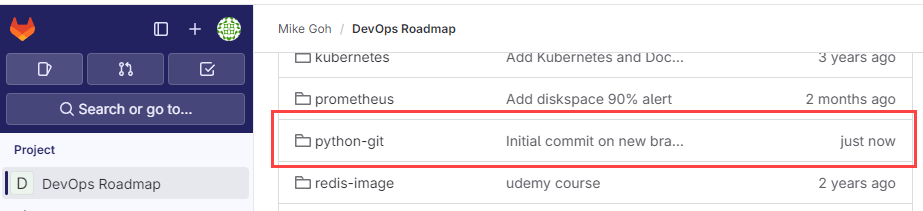
Conclusion
Hence, we have a concise Python script that locates the git root directory and automatically commits and pushes new development codes to a designated branch. With a click of a button from my online web form, the update-to-date playbook and inventory files are made available for the Ansible Tower project to sync with the associated Git repository. There are likely more effective methods, such as utilizing VS Code plugins or Jenkins, wherein modified codes are automatically pushed on a file save. However, my code snippets are intended to be embedded within the Python web form that I have created.